Should You Use "border: none" or "border: 0" in CSS?
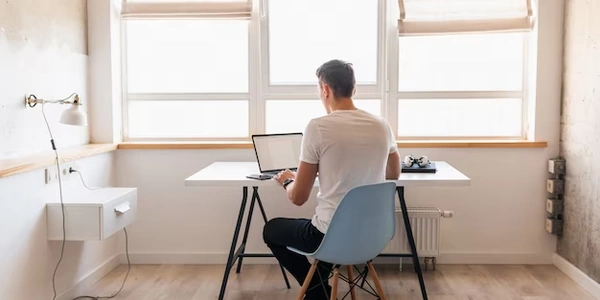
I'd be glad to explain the CSS code for removing borders:
border: none vs. border: 0
In CSS, both border: none
and border: 0
achieve the same outcome: they remove the border from an element. However, there are some subtle differences to consider:
-
Technical Specificity:
border: none
is the more specific way according to the CSS specification. It directly sets theborder-style
property tonone
, indicating the absence of any border style (solid, dashed, dotted, etc.).border: 0
is a shorthand that sets all individual border properties (border-width
,border-style
, andborder-color
) to 0. While it achieves the same visual effect, it's less specific.
-
Readability and Maintainability:
- Some developers prefer
border: none
for its clarity, explicitly stating the removal of the border style. - Others favor
border: 0
for its conciseness, especially when dealing with multiple border properties (e.g.,border-top: 0; border-right: 0;
).
- Some developers prefer
-
Performance (Negligible Difference):
Recommendation:
- Clarity: If you prioritize code readability, use
border: none
. - Conciseness: If brevity is your main concern,
border: 0
is perfectly acceptable.
Ultimately, both approaches are valid. Choose the one that aligns best with your coding style and project conventions.
Additional Notes:
- If you only need to remove a specific border side (top, right, bottom, or left), you can use properties like
border-top: 0
. - To reset all borders to their default state (usually a thin gray border), use
border: inherit
.
I hope this explanation clarifies the use of border: none
and border: 0
in CSS!
Here are some example codes demonstrating how to remove borders with both border: none
and border: 0
in CSS:
Removing all borders:
/* Using border: none */
.no-border {
border: none;
}
/* Using border: 0 (shorthand) */
.no-border-shorthand {
border: 0;
}
Removing a specific border side (top):
.no-top-border {
border-top: none; /* Using border-top: none */
}
.no-top-border-shorthand {
border-top: 0; /* Using border-top: 0 (shorthand) */
}
Resetting all borders to default:
.default-border {
border: inherit;
}
These examples showcase the different approaches you can take to manage borders in your CSS. You can choose the method that best suits your needs and coding preferences.
While border: none
and border: 0
are the most straightforward ways to remove borders in CSS, there are a few alternative methods that can be useful in specific situations:
Using outline: none (for focus indicators):
- This approach targets the outline that appears around an element when it receives focus (e.g., when clicked or tabbed to).
- It's particularly useful for interactive elements like buttons and links to create a cleaner, more custom focus style.
button {
border: none; /* Remove default border */
outline: none; /* Remove default focus outline */
}
Resetting Borders with box-sizing: border-box:
- This technique doesn't directly remove borders, but it can be helpful when dealing with padding and borders.
- By default, CSS uses the
content-box
model, where padding and border widths add to the element's overall dimensions. - Setting
box-sizing: border-box
makes the padding and border sit within the declared width and height of the element, giving you more control over the element's final size.
.element {
width: 200px; /* Declared width */
height: 100px; /* Declared height */
padding: 10px; /* Padding will fit within dimensions */
border: 1px solid #ddd; /* Border will fit within dimensions */
box-sizing: border-box;
}
Using overflow: hidden (with caution):
- This approach is generally not recommended for border removal as it can have unintended consequences.
overflow: hidden
clips the content of the element, potentially hiding parts of the element that extend beyond its declared dimensions, including borders.- While it might seem like it removes the border, it's actually cutting it off. Avoid this method unless you understand its limitations and potential accessibility issues.
Choosing the Right Method:
- For basic border removal,
border: none
orborder: 0
are the best options. - Use
outline: none
to customize focus indicators. - Employ
box-sizing: border-box
when you need precise control over element dimensions with padding and borders. - Avoid
overflow: hidden
for border removal due to potential issues.
css border
Unlocking Clean Code: Separating Content and Presentation in HTML and CSS
Separation of Concerns:HTML defines the structure and content of a webpage. Tables are meant to present data in a tabular format...
Making Your Div Reach the Top: A Guide to 100% Height in HTML and CSS
HTML Structure:The basic HTML structure involves a div element that you want to control the height of. You can place this div wherever you need it in your HTML document...
Styling HTML Tables: Alternate Row Background Colors with CSS
I'd be glad to explain how to achieve alternate table row colors using CSS for HTML tables:Understanding the Goal:In HTML tables...
The Superhero Family of Sass: SCSS and Original Sass
In a nutshell:Sass is the umbrella term for the entire ecosystem, including the language itself and its features. Think of it as the whole superhero family...